9. Tensor¶
9.1. what’s tensor¶
Very briefly, a tensor is an N-dimensional array containing the same type of data (int32, bool, etc.): All you need to describe a tensor fully is:
- its data type and
- the value of each of the N dimension.
9.2. class Tensor¶
In tf python API, Tensor is a class, <class ‘tensorflow.python.framework.ops.Tensor’>. The data flowing in the graph are instance of Tensor.
从class Tensor的 官方文档, 可得对于tensor的理解,见如下代码的注释:
1 2 3 4 5 6 7 8 | #c, d, and e are Tensor objects, a symbolic(象征性的) handle to one of the outputs of an Operation.
#c, d, and e do not hold the values of that operation's output, but build a dataflow connection between operations
c = tf.constant([[1.0, 2.0], [3.0, 4.0]])
d = tf.constant([[1.0, 1.0], [0.0, 1.0]])
e = tf.matmul(c, d)
#如果要得到“the value that `e` represents”,就得Execute the graph
sess = tf.Session() # Construct a `Session` to execute the graph.
result = sess.run(e) #result就不是Tensor对象了,而是numpy.ndarray对象
|
9.4. tensor name¶
按照一定的规则,由 operation’s name 来决定。
A tensor name has the form “<OP_NAME>:<i>” where:
- “<OP_NAME>” is the name of the operation that produces it.
- “<i>” is an integer representing the index of that tensor among the operation’s outputs.
9.5. Tensor-like objects¶
tf中有5种 tensor-like objects ,它们在进入operation进行计算时,都将被转换为tf.Tensor
9.6. Manipulate the Shape¶
9.6.1. The shape of numpy’s array¶
https://stackoverflow.com/questions/22053050/difference-between-numpy-array-shape-r-1-and-r
9.6.2. tensor shape and tensor rank¶
rank | dimension | 数学实例 | python例子 | shape |
0 | 0-D | 纯量 (只有大小) | s = 483 | [] |
1 | 1-D | 向量(大小和方向) | v = [1.1, 2.2, 3.3] | [3] |
2 | 2-D | 矩阵(数据表) | m = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] | [3,3] |
9.6.4. Change the Tensor Shape¶
- tf.reshape()
- tf.squeeze(),把tensor的shape中值为1的维度去掉
9.6.5. 返回tensor’s shape¶
1 2 | t = tf.constant([[[1, 1, 1], [2, 2, 2]], [[3, 3, 3], [4, 4, 4]]])
tf.shape(t) # [2, 2, 3]
|
9.6.6. 返回tensor’s rank¶
1 2 3 | # shape of tensor 't' is [2, 2, 3]
t = tf.constant([[[1, 1, 1], [2, 2, 2]], [[3, 3, 3], [4, 4, 4]]])
tf.rank(t) # 3
|
9.7. Manipulate the Type¶
9.8. Manipulate the Element¶
9.8.1. 获得tensor某个维度上的最值的index¶
1 | tf.argmax(input_tensor, axis)
|
9.8.2. 从Tensor中摘取数据¶
- tf.gather(),从一个tensor(params)的指定维度(axis)的指定位置(indices)获取element,组合成一个tensor
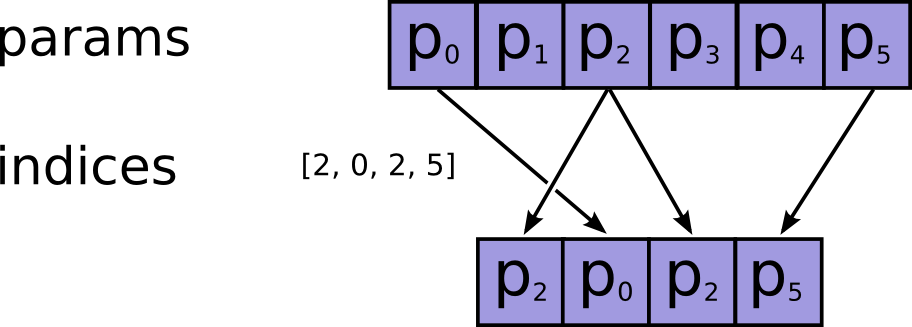
- tf.where(
- condition, x=None, y=None, name=None),从两个tensor,x,y,中选取element/row,组合成一个tensor
9.8.4. 矩阵按元素相乘¶
1 | matrix_1 * matrix_2
|
9.8.5. 矩阵按行/列求和¶
1 | tf.reduce_sum(matrix, axis)
|
其中,
- axis=0, 按列
- axis=1, 按行
- axis=None, all dimensions are reduced, and a tensor with a single element is returned.
9.8.6. element-wise 比较两个tensor¶
1 2 3 4 5 | equal(
x,
y,
name=None
)
|
9.9. 求tensor的值(Evaluating)¶
Evaluating tensor和executing a graph是一个意思。
1 2 3 4 5 6 7 8 9 | >>> a = tf.constant([10,20])
>>> a
<tf.Tensor 'Const_1:0' shape=(2,) dtype=int32>
>>> sess = tf.InteractivateSession()
>>> v = sess.run(a)
>>> v
array([10, 20])
>>> type(v)
<class 'numpy.ndarray'>
|